基于安卓Android社会百态新闻系统APP的设计与实现(MySQL)(含录像)(毕业论文7900字,安卓APP客户端和JAVA服务端程序代码,MySQL数据库)
该系统的客户端定位于Android手机平台。通过对聊天软件的调研,进行需求分析、总体设计、UI设计、数据库设计,利用Java技术在Android平台上实现聊天软件的各个功能模块,设计测试用例,调试并完善系统功能。
新闻模块
各个分类(各类新闻,娱乐八卦,社会要闻,军事信息等)的新闻,开题报告,实时更新。
图片模块
可以上传图片,浏览观看图片。
视频模块
个人可以上传视频,浏览观看视频。权限、个人会员和管理员
会员中心模块
对登陆的信息进行基本操作,开题报告,更换头像修改密码,签到,查询等。可以收藏图片或者视频。向管理员反馈信息。
管理员模块
管理全部信息。并可以接受会员反馈信息。
开发环境:Eclipse
数据库:MySQL
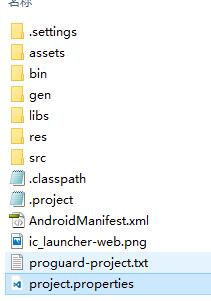
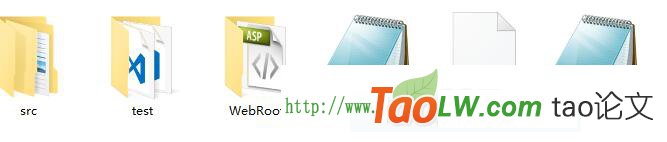
package com.szy.news.service;
import java.util.ArrayList;
import java.util.List;
import org.apache.http.HttpResponse;
import org.apache.http.HttpStatus;
import org.apache.http.client.HttpClient;
import org.apache.http.client.entity.UrlEncodedFormEntity;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.impl.client.DefaultHttpClient;
import org.apache.http.message.BasicNameValuePair;
import org.apache.http.params.BasicHttpParams;
import org.apache.http.params.HttpConnectionParams;
import org.apache.http.params.HttpParams;
import org.apache.http.protocol.HTTP;
import org.apache.http.util.EntityUtils;
import com.szy.news.model.Parameter;
/**
*@author coolszy
*@date 2012-3-26
*@blog http://blog.92coding.com
*
*以同步方式发送Http请求
*/
public class SyncHttp
{
/**
* 通过GET方式发送请求
* @param url URL地址
* @param params 参数
* @return
* @throws Exception
*/
public String httpGet(String url, String params) throws Exception
{
String response = null; //返回信息
//拼接请求URL
if (null!=params&&!params.equals(''))
{
url += '?' + params;
}
int timeoutConnection = 3000;
int timeoutSocket = 5000;
HttpParams httpParameters = new BasicHttpParams();// Set the timeout in milliseconds until a connection is established.
HttpConnectionParams.setConnectionTimeout(httpParameters, timeoutConnection);// Set the default socket timeout (SO_TIMEOUT) // in milliseconds which is the timeout for waiting for data.
HttpConnectionParams.setSoTimeout(httpParameters, timeoutSocket);
// 构造HttpClient的实例
HttpClient httpClient = new DefaultHttpClient(httpParameters);
// 创建GET方法的实例
HttpGet httpGet = new HttpGet(url);
try
{
HttpResponse httpResponse = httpClient.execute(httpGet);
int statusCode = httpResponse.getStatusLine().getStatusCode();
if (statusCode == HttpStatus.SC_OK) //SC_OK = 200
{
// 获得返回结果
response = EntityUtils.toString(httpResponse.getEntity());
}
else
{
response = '返回码:'+statusCode;
}
} catch (Exception e)
{
throw new Exception(e);
}
return response;
}
/**
* 通过POST方式发送请求
* @param url URL地址
* @param params 参数
* @return
* @throws Exception
*/
public String httpPost(String url, List<Parameter> params) throws Exception
{
String response = null;
int timeoutConnection = 3000;
int timeoutSocket = 5000;
HttpParams httpParameters = new BasicHttpParams();// Set the timeout in milliseconds until a connection is established.
HttpConnectionParams.setConnectionTimeout(httpParameters, timeoutConnection);// Set the default socket timeout (SO_TIMEOUT) // in milliseconds which is the timeout for waiting for data.
HttpConnectionParams.setSoTimeout(httpParameters, timeoutSocket);
// 构造HttpClient的实例
HttpClient httpClient = new DefaultHttpClient(httpParameters);
HttpPost httpPost = new HttpPost(url);
if (params.size()>=0)
{
//设置httpPost请求参数
httpPost.setEntity(new UrlEncodedFormEntity(buildNameValuePair(params),HTTP.UTF_8));
}
//使用execute方法发送HTTP Post请求,并返回HttpResponse对象
HttpResponse httpResponse = httpClient.execute(httpPost);
int statusCode = httpResponse.getStatusLine().getStatusCode();
if(statusCode==HttpStatus.SC_OK)
{
//获得返回结果
response = EntityUtils.toString(httpResponse.getEntity());
}
else
{
response = '返回码:'+statusCode;
}
return response;
}
/**
* 把Parameter类型集合转换成NameValuePair类型集合
* @param params 参数集合
* @return
*/
private List<BasicNameValuePair> buildNameValuePair(List<Parameter> params)
{
List<BasicNameValuePair> result = new ArrayList<BasicNameValuePair>();
for (Parameter param : params)
{
BasicNameValuePair pair = new BasicNameValuePair(param.getName(), param.getValue());
result.add(pair);
}
return result;
}
}
package com.szy.news.model;
/**
*@author coolszy
*@date 2012-3-26
*@blog http://blog.92coding.com
*/
public class Category
{
//类型编号
private int cid;
//类型名称
private String title;
//类型次数
private int sequnce;
public Category()
{
super();
}
public Category(int cid, String title)
{
super();
this.cid = cid;
this.title = title;
}
public Category(int cid, String title, int sequnce)
{
super();
this.cid = cid;
this.title = title;
this.sequnce = sequnce;
}
public int getCid()
{
return cid;
}
public void setCid(int cid)
{
this.cid = cid;
}
public String getTitle()
{
return title;
}
public void setTitle(String title)
{
this.title = title;
}
public int getSequnce()
{
return sequnce;
}
public void setSequnce(int sequnce)
{
this.sequnce = sequnce;
}
@Override
public String toString()
{
return title;
}
}
package com.szy.news.model;
import java.io.Serializable;
/**
*@author coolszy
*@date 2012-3-26
*@blog http://blog.92coding.com
*/
public class Parameter implements Serializable, Comparable<Parameter>
{
private static final long serialVersionUID = 2721340807561333705L;
private String name;//参数名
private String value;//参数值
public Parameter()
{
super();
}
public Parameter(String name, String value)
{
super();
this.name = name;
this.value = value;
}
public String getName()
{
return name;
}
public void setName(String name)
{
this.name = name;
}
public String getValue()
{
return value;
}
public void setValue(String value)
{
this.value = value;
}
@Override
public String toString()
{
return 'Parameter [name=' + name + ', value=' + value + ']';
}
@Override
public boolean equals(Object arg0)
{
if (null == arg0)
{
return false;
}
if (this == arg0)
{
return true;
}
if (arg0 instanceof Parameter)
{
Parameter param = (Parameter) arg0;
return this.getName().equals(param.getName()) && this.getValue().equals(param.getValue());
}
return false;
}
@Override
public int compareTo(Parameter param)
{
int compared;
compared = name.compareTo(param.getName());
if (0 == compared)
{
compared = value.compareTo(param.getValue());
}
return compared;
}
}